Set up push notifications
UpdatedThe Expo plugin supports push notifications over APN for iOS and FCM for Android. Use this page to get started with push notification services.
How it works
When you use our Expo plugin, you’re already set up to support push notifications, including images and deep links.
Before a device can receive a push notification, you must:
- Identify a person. This associates a token with the person; you can’t send push notifications to a device until you identify the recipient.
- (Optional) Set up your app to report push metrics back to Customer.io.
Before you begin
You need to enable Customer.io to send push notifications through your preferred service: Apple Push Notification Service (APNs) and/or Firebase Cloud Messaging (FCM) before you can send push notifications through Customer.io.
We don’t support Expo’s Push Service
You can’t use push tokens obtained through Expo’s Push Service to send push notifications through Customer.io. If your app previously used Expo’s push service, you’ll have to obtain new FCM or APN tokens to send push notifications through Customer.io.
Luckily, you don’t need to do anything to support your app’s users when you implement our SDK! If your app can obtain Expo push tokens, it should also be set up to receive APN or FCM device tokens. You’ll simply configure your app to fetch a token and send that token to Customer.io using CustomerIO.registerDeviceToken("<apn or fcm token here>")
.
Set up push for Android
You don’t need to do anything to support Android devices. When you use our Expo plugin, your Android audience is automatically set up to receive push notifications.
Set up push on iOS
For the most part, we’ll set up push notifications for you when you run expo prebuild
. But before you can support push notifications for iOS devices, you need to add Push Notification capabilities to your project in XCode.
- Open your React Native project in XCode, go to the
ios
subfolder and open<yourAppName>.xcworkspace
. - Select your project, and then under Targets, select your main app.
- Click the Signing & Capabilities tab
- Click Capability.
- Add Push Notifications to your app.
When you’re done, you’ll see Push Notifications added to your app’s capabilities. Now you’re ready to run the expo prebuild
.
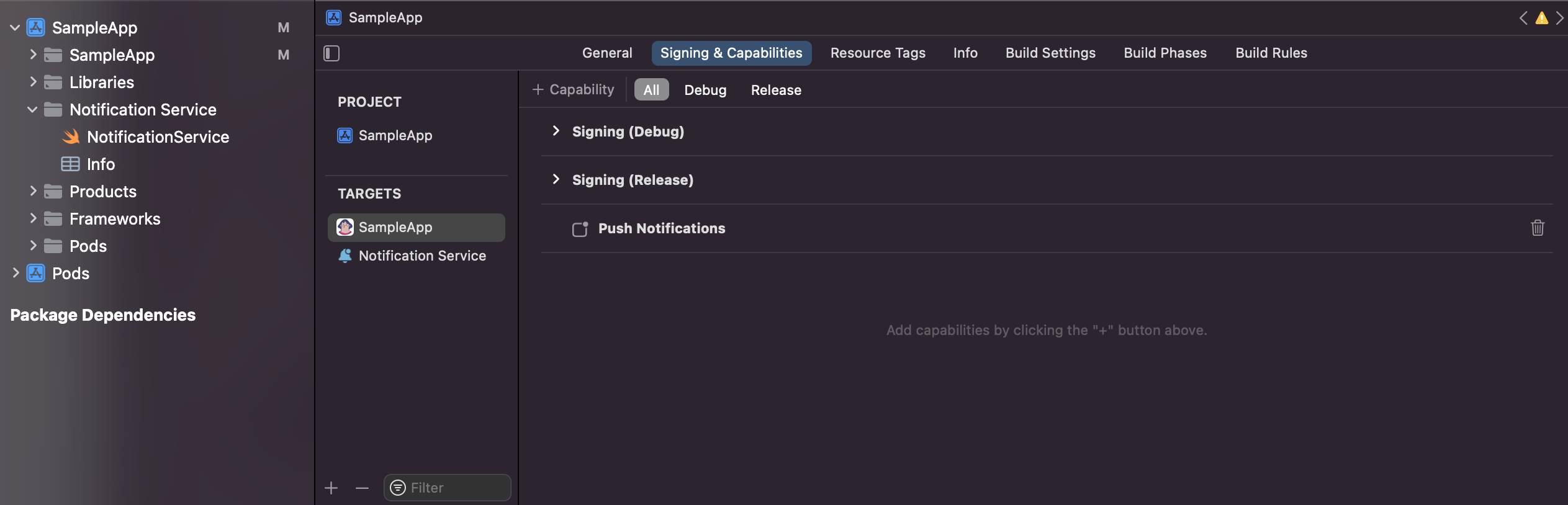
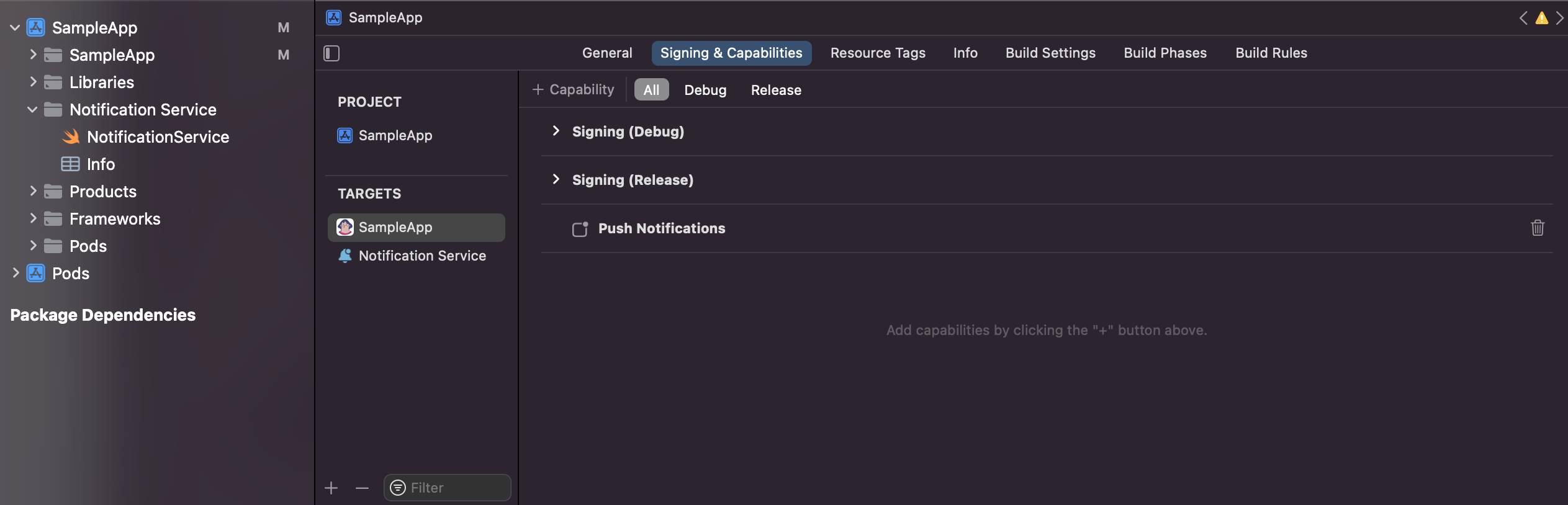
Update iOS dependencies
In some cases, we may make fixes in our iOS push packages that fix downstream issues in the Expo plugin. The command to update these packages depends on whether your Expo project uses a managed or bare workflow. For managed workflows, you can simply re-run the prebuild.
EAS Build with Rich Push
If you use EAS Build with useRichPush: true
, the Customer.io plugin automatically creates a notification service extension during expo prebuild
. You need to tell EAS Build about this extension by adding the ios.appExtensions
configuration to your app.json
or app.config.js
:
{
"expo": {
"ios": {
"bundleIdentifier": "com.yourcompany.yourapp",
"appExtensions": [
{
"targetName": "NotificationService",
"bundleIdentifier": ${bundleIdentifier} + ".richpush", // e.g. com.yourcompany.yourapp.richpush
"entitlements": {
"com.apple.developer.usernotifications.service": true
}
}
]
}
}
}
Finding the extension bundle identifier: After running expo prebuild
, you can find the exact bundle identifier in your ios/<YourAppName>.xcworkspace
project. Open the project in Xcode, look for the NotificationService
target, and check its bundle identifier in the project settings. It will be your main app’s bundle identifier with .richpush
appended.
Sound in push iOS push notifications
When you send a push notification to iOS devices that uses our SDK, you can opt to send the Default system sound or no sound at all. If your audience’s phone is set to vibrate, or they’ve disabled sound permissions for your app, the Default setting will cause the device to vibrate rather than playing a sound.
In most cases, you should use the Default sound setting to make sure your audience hears (or feels) your message. But, before you send sound, you should understand:
- Your app needs permission from your users to play sounds. This is done by your app, not our SDKs. Here’s an example from our iOS sample app showing how to request sound permissions.
- iOS users can go into System Settings and disable sound permissions for your app. Enabling the Default setting doesn’t guarantee that your audience hears a sound when your message is delivered!
We don’t support custom sounds yet
If you want to send a custom sound, you’ll need to handle it on your own, outside the SDK and use a custom payload when you set up your push notifications.
Prompt users to opt-into push notifications
Your audience has to opt into push notifications. To display native iOS and Android push notification permission prompts, you’ll use the CustomerIO.pushMessaging.showPromptForPushNotifications
method.
You can configure push notifications to request authorization for sounds and badges as well (only on iOS). If a user opts into push notifications, the CustomerIO.pushMessaging.showPromptForPushNotifications
method will return Granted
, otherwise it returns Denied
as a string
. If the user already responded to the push authorization prompt, the current authorization status is returned as a string.
var options = {"ios" : {"sound" : true, "badge" : true}}
CustomerIO.showPromptForPushNotifications(options).then(status => {
switch(status) {
case "Granted":
// Push permission is granted, your app can now receive push notifications
break;
case "Denied":
// App is not authorized to receive push notifications
// You might need to explain users why your app needs permission to receive push notifications
break;
case "NotDetermined":
// Push permission status is not determined (Only for iOS)
break;
}
}).catch(error => {
// Failed to show push permission prompt
console.log(error)
})
Get a user’s permission status
To get a user’s current permission status, call the CustomerIO.getPushPermissionStatus()
method. This returns a promise with the current status as a string.
CustomerIO.getPushPermissionStatus().then(status => {
console.log("Push permission status is - " + status)
})
Fetch the current device token
You can fetch the currently stored device token using the CustomerIO.pushMessaging.getRegisteredDeviceToken()
method. This method returns an APN/FCM token in a promise as a string.
let token = await CustomerIO.pushMessaging.getRegisteredDeviceToken()
if (token) {
// Use the token as required in your app for example save in a state
setDeviceToken(token);
}
Test your implementation
After you set up rich push, you should test your implementation. Below, we show the payload structure we use for iOS and Android. In general, you can use our regular rich push editor; it’s set up to send messages using the JSON structure we outline below.
If you want to fashion your own payload, you can use our custom payload.
iOS APNs payload
{
"aps": {
// basic iOS message and options go here
"mutable-content": 1,
"alert": {
"title": "string", //(optional) The title of the notification.
"body": "string" //(optional) The message you want to send.
}
},
"CIO": {
"push": {
"link": "string", //generally a deep link, i.e. my-app:://...
"image": "string" //HTTPS URL of your image, including file extension
}
}
}
-
-
- image stringThe URL of an HTTPS image that you want to use for your message.
- link stringA deep link (to a page in your app), or a link to a web page.
-
-
- alertstringA simple alert message.
- badge integerThe number you want to display on your app’s icon. Set to 0 to remove the current badge, if any.
- category stringThe notification’s type. This string must correspond to the identifier of one of the
UNNotificationCategory
objects you register at launch time. - content-available integerThe background notification flag. Use
1
without analert
to perform a silent update.0
indicates a normal push notification. - interruption-level stringIndicates the importance and delivery timing of a notification.
Accepted values:
passive
,active
,time-sensitive
,critical
- mutable-content integerIf you use the Customer.io SDK, you must set this value to
1
to support images and “delivered” metrics from your push notifications. When the value is 1, your notification is passed to your notification service app extension before delivery. Use your extension to modify the notification’s content. - relevance-score numberA number between 0 and 1. The highest score is considered the “most relevant” and is featured in the notification summary.
- soundstringThe name of a sound file in your app’s main bundle or in the Library/Sounds folder of your app’s container directory. Use “default” to play the system sound. For critical alerts, you’ll pass an object instead.
- target-content-id stringThe identifier of the window brought forward.
- thread-id stringAn identifier to group related notifications.
Android payload
{
"message": {
"data": {
"title": "string", //(optional) The title of the notification.
"body": "string", //The message you want to send.
"image": "string", //https URL to an image you want to include in the notification
"link": "string" //Deep link in the format remote-habits://deep?message=hello&message2=world
}
}
}
- messageRequired The parent object for all push payloads.
-
-
- body_loc_arg stringVariable string values used in place of the format specifiers in
body_loc_key
to localize the body text to the user’s current localization. See Formatting and Styling for more information. - body_loc_key stringThe key to the body string in the app’s string resources that you want to use to localize the body text to the user’s current localization. See String Resources for more information.
- click_action stringThe action that occurs when a user taps on the notification. Launches an activity with a matching intent filter when a person taps the notification.
- color stringThe notification’s icon color in
#rrggbb
format. - icon stringSets the notification icon to
myicon
for drawable resourcemyicon
. If you don’t send this key, FCM displays the launcher icon from your app manifest. - sound stringThe sound that plays when the device receives the notification. Supports
"default"
or the filename of a sound resource bundled in your app. Sound files must reside in/res/raw/
. - tag string
Identifier to replace existing notifications in the notification drawer. If empty, each request creates a new notification.
If you specify a tag, and a notification with the same tag is already being shown, the new notification replaces the existing one in the notification drawer.
- title_loc_arg stringVariable string values used in place of the format specifiers in
title_loc_key
to localize the title text to the user’s current localization. See Formatting and Styling for more information. - title_loc_key stringThe key to the title string in the app’s string resources that you want to use to localize the title text to the user’s current localization. See String Resources for more information.
-
-
- body stringThe body of your push notification.
- image stringThe URL of an HTTPS image that you want to use for your message.
- link stringA deep link (to a page in your app), or a link to a web page.
- title stringThe title of your push notification.
-