Quick Start Guide
UpdatedBefore you can take advantage of our SDK, you need to set up your development environment, install the SDK, and then initialize it. This page can help you get started!
1. Set up your development environment
Before you get started, you’ll need to do the following things:
- Set up your React Native environment
- Add React navigation to your project to support deep links and screen tracking
- Set up your iOS environment:
- Setup XCode (using deployment target to 13.0 or later).
- Make sure that you have XCode command line tools installed
xcode-select --install
. - Get your Apple Push Certificate and enable push notifications for iOS in your Customer.io account.
- Have an iOS 13 to test with. You cannot test push notifications in an emulator.
- Set up your Android environment:
- Download and install Android Studio
- Add your Google Firebase Cloud Messaging (FCM) key to Customer.io and enable push notifications for Android
- Make sure you use an appropriate version of the Android Gradle plugin.
- Have an Android device or emulator with Google Play Services enabled and a minimum OS version.
2. Create a React Native source Source
Before you can integrate your app, you need to tell Customer.io that you have an React native app. This is what we call a sourceA source is a website or server that you want to capture data from—it’s a source of data!.
Your source provides the API key you’ll use to initialize the SDK.
- Go to the Data Pipelines tab. On the Connections page under Sources, click Add Source.
- Select the Mobile: React Native source and then click Next: Connect React Native.
- Enter a Name for the source, like “My React Native App”.
- We’ll present you with a code sample containing a
cdpApiKey
that you’ll use to initialize the SDK. Copy this key and keep it handy. - Click Complete Setup to finish setting up your source.
Now the Connections page shows that your React Native source is connected to your Journeys workspace. Hover over a source or destination to see its active connections. You can also connect your React Native source to additional destinations if you want to send your mobile data to additional services—like your analytics provider, data warehouse, or CRM.
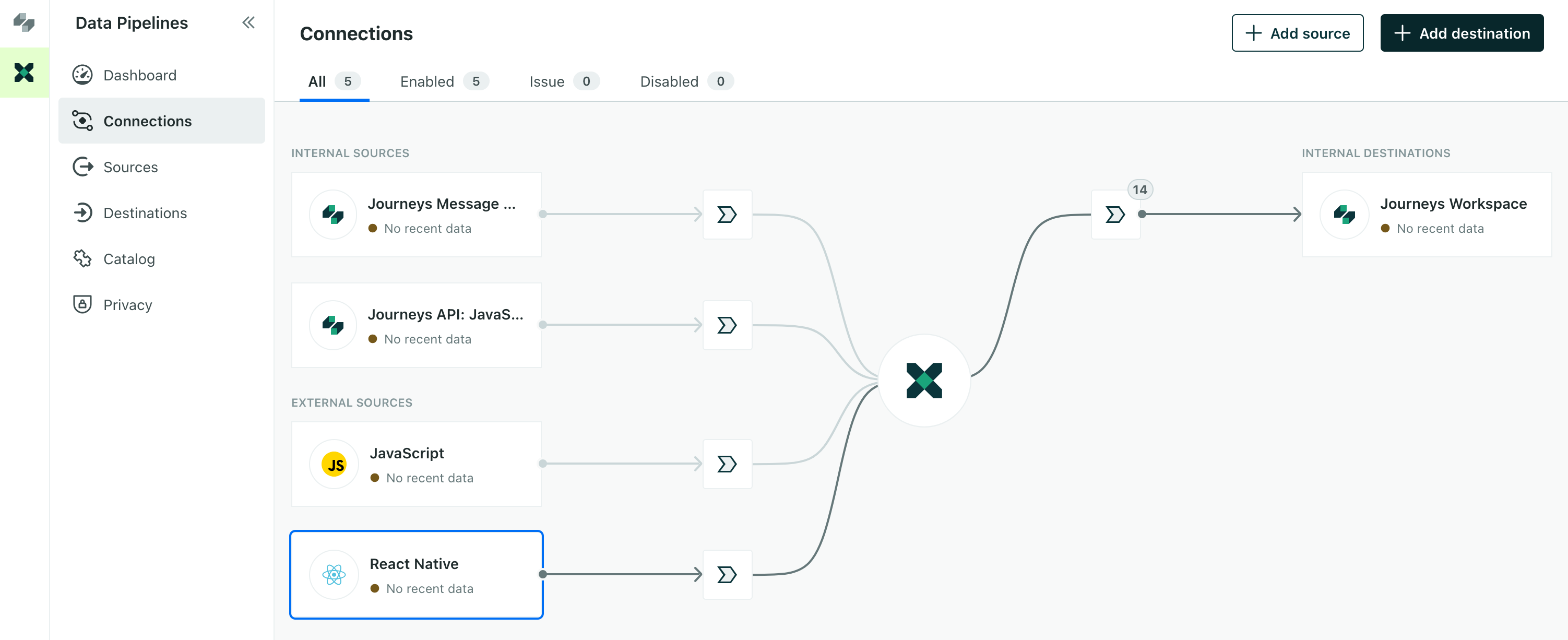
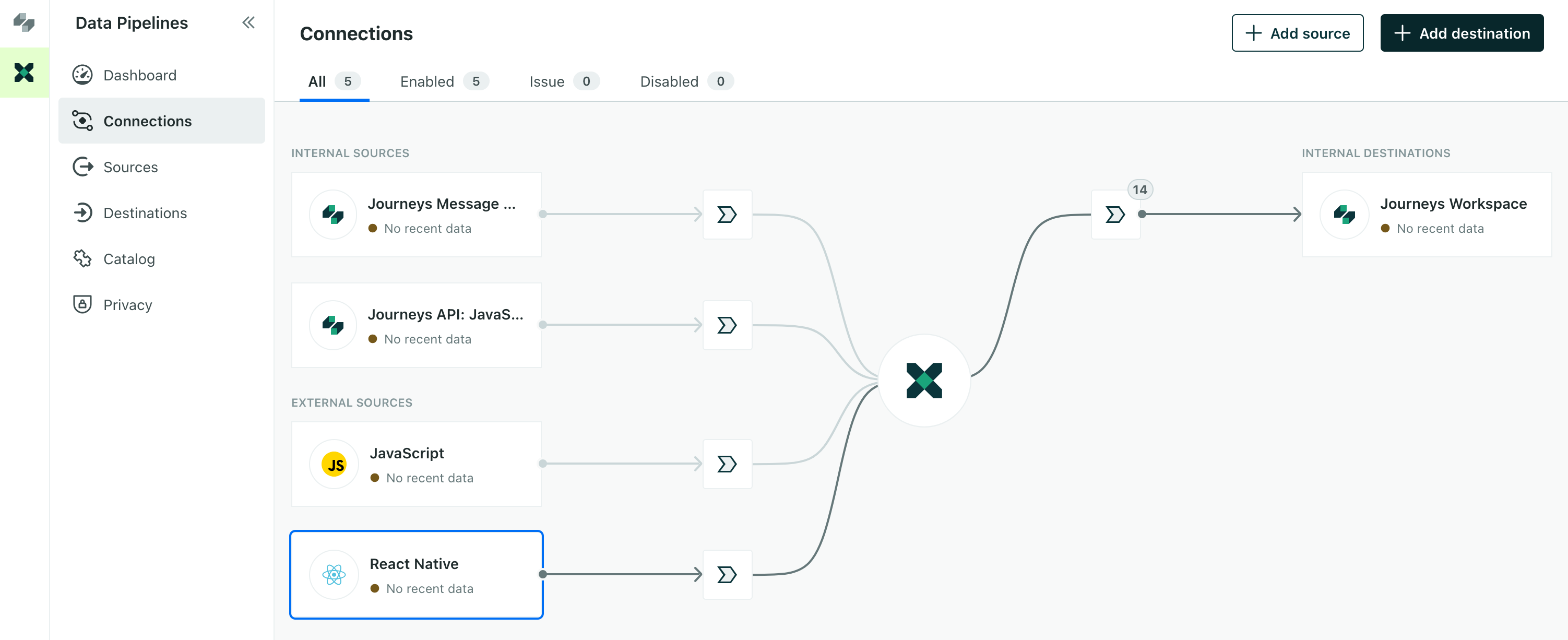
3. Install the React Native SDK
Now it’s time to add the React Native packages to your app and install everything.
Open your terminal and go to your project folder—
cd <Root/path/to/your/app>
.Install the
customerio-reactnative
package using NPM or Yarn:npm install customerio-reactnative
yarn add customerio-reactnative
If you’re using a React Native version earlier than 0.60, link the library manually with
npx react-native link customerio-reactnative
. Otherwise, go to the next step.In your terminal, run
pod install --repo-update --project-directory=ios
. This adds the required iOS dependencies to your project. When the process is complete , you’ll see a message like this:Pod installation complete! There are X dependencies from the Podfile and Y total pods installed.
Make sure that your minimum deployment target is set to
13.0
. You’ll have to do this in two places:Go to the Android subfolder and include google-services-plugin by adding the following lines to the project-level
android/build.gradle
file:buildscript { repositories { // Add this line if it isn't already in your build file: google() // Google's Maven repository } dependencies { // Add this line: classpath 'com.google.gms:google-services:<version-here>' // Google Services plugin } } allprojects { repositories { // Add this line if it isn't already in your build file: google() // Google's Maven repository } }
Add the following line to
android/app/build.gradle
:apply plugin: 'com.google.gms.google-services' // Google Services plugin
Download
google-services.json
from your Firebase project and copy the file toandroid/app/google-services.json
.
Now you’re ready to initialize the SDK and use it in your app.
4. Initialize the SDK
After you install the SDK, you’ll need to initialize it in your app. To do this, you’ll add initialization code in your App.js
file—or wherever you want to initialize the customerio-reactnative
package.
You’ll need two different keys to initialize the SDK. See Authentication you don’t have them, or don’t know where to find them.
- Your sourceA source is a website or server that you want to capture data from—it’s a source of data! CDP API Key, which you got when you created your react native source in earlier steps.
- Your Site IDEquivalent to the user name you’ll use to interface with the Journeys Track API; also used with our JavaScript snippets. You can find your Site ID under Settings > Workspace Settings > API Credentials to initialize the in-app package. You can find your
siteId
in Customer.io under Settings > Workspace Settings > API Credentials.
import React, {useEffect} from 'react';
import {
CioLogLevel, CioRegion, CustomerIO, CioConfig
} from 'customerio-reactnative';
const App = () => {
useEffect(() => {
const config: CioConfig = {
cdpApiKey: 'CDP API Key', // Mandatory
migrationSiteId: 'siteId', // Required if migrating from an earlier version
region: CioRegion.US,
logLevel: CioLogLevel.Debug,
trackApplicationLifecycleEvents: true,
inApp: {
siteId: 'site_id',
},
push: {
android: {
pushClickBehavior: PushClickBehaviorAndroid.activityPreventRestart
}
}
};
CustomerIO.initialize(config)
}, [])
}
When you’re done, you might want to return to your main folder and run your application to make sure that everything’s set up correctly:
- iOS:
npx react-native run-ios
- Android:
npx react-native run-android
Check out our code samples!
You’ll find a complete, working sample apps in our React Native SDK’s App
directory. If you get stuck, you can refer to the sample apps to see how everything fits together.